Flutter 入门学习之布局组件
基本布局
Container
Container 可以设置固定的宽高属性,没有其他参数设置时,Container将会根据子控件自行调整大小:
1 | Container( |
其次,还可以通过constraints
属性设置最大/小宽、高来确定大小,constraints 如果不设置,默认最小宽高是0,最大宽高是无限大(double.infinity):
1 | Container( |
注意:设置对齐方式后,Container 将会充满其父控件,相当于 Android 中 match_parent,不在是根据子控件调整大小
通过 transform
可以旋转、平移、缩放Container:
1 | Container( |
Flexible、Expanded和 Spacer 都是具有权重属性的组件,可以控制 Row、Column、Flex 的子控件如何布局的控件。
Flexible
Flexible组件可以使Row、Column、Flex等子组件在主轴方向有填充可用空间的能力(例如,Row在水平方向,Column在垂直方向),但是它与 Expanded 组件不同,它不强制子组件填充可用空间。
构造函数:
1 | class Flexible extends ParentDataWidget<Flex> { |
Flexible 中flex
参数表示子控件的占比,fit
参数表示填满剩余空间的方式,说明如下:
- tight:必须(强制)填满剩余空间。
- loose:尽可能大的填满剩余空间,但是可以不填满。
比如,Column 中有 4 个子控件,上下的固定宽,中间的占满剩余的空间,且按比例分布,代码如下:
1 | Column( |
对于 fit
参数是针对于 类似 Container 的这样的组件而言的,Container 默认是适配子控件大小的,但当设置对齐方式时 Container 将会填满父控件,因此是否填满剩余空间取决于子控件是否需要填满父控件。
也就是在,没有设置对其方式时,可以通过 fit
参数的 tight 强制使其填满父布局的大小,看下面三个例子即可:
1 | Row( |
Expanded
Expanded继承字Flexible,fit
参数固定为FlexFit.tight
,也就是说Expanded必须(强制)填满剩余空间。
构造方法:
1 | class Expanded extends Flexible { |
Spacer
其源代码:
1 | class Spacer extends StatelessWidget { |
Spacer 的通过 Expanded 的实现的,和 Expanded 的区别是:Expanded可以设置子控件,而 Spacer 的子控件尺寸是0,因此 Spacer 适用于撑开Row、Column、Flex的子控件的空隙,可以将剩余的空间按比例划分,用法如下:
1 | Row( |
层叠布局
Stack
Stack组件可以将子组件叠加显示,对于子控件的顺序,则是第一个被绘制在最底端,后面的依次在上一个控件的上面,如果想调整显示的顺序,则可以通过摆放子控件的顺序来进行。
1 | Stack({ |
Stack未定位的子组件大小由fit
参数决定,默认值是StackFit.loose
,表示子组件自己决定,StackFit.expand
表示尽可能的大,是否定位取决于是否为Positioned
子组件:
- Positioned的子组件,它们的位置会根据所设置的top、bottom、right以及left属性来确定,这几个值都是相对于Stack的左上角;
- 对于非Positioned的子组件,它们会根据Stack的 aligment 来设置位置。
示例代码:
1 | Stack( |
IndexedStack
IndexedStack是Stack的子类,Stack是将所有的子组件叠加显示,而 IndexedStack 只显示 index 指定的子组件,即第第 index 个子组件。
定位和对齐
Align & Center
创建一个位置对齐的组件,默认对齐方式居中[
Alignment.center
]。
1 | const Align({ |
alignment
:传入一个 AlignmentGeometry 对象,其为抽象类,子类有 Alignment 和 AlignmentDirectional,以及Alignment的子类 FractionalOffset;widthFactor
&heightFactor
:非空则将 Align 的宽度 / 高度设置为该因子的倍乘。
代码示例
1 | Scaffold( |
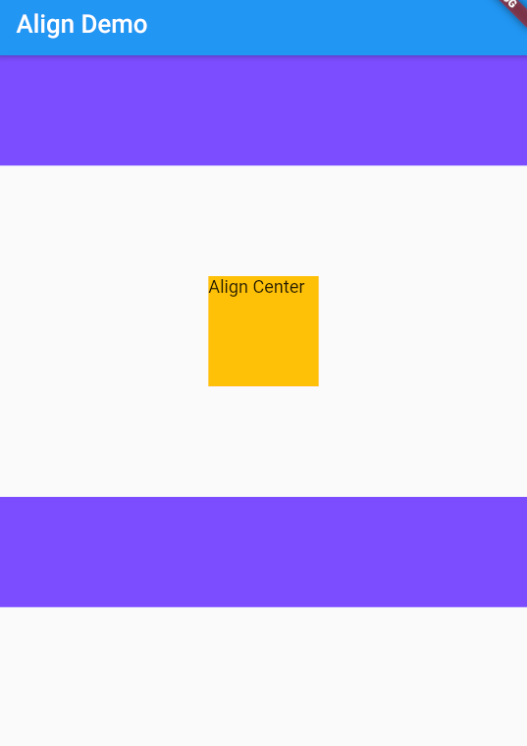
Center 控件继承自 Align,默认子组件居中:
1 | class Center extends Align { |